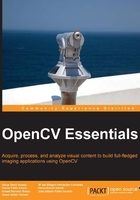
上QQ阅读APP看书,第一时间看更新
Reading and playing a video file
A video deals with moving images rather than still images, that is, display of a frame sequence at a proper rate (FPS or frames per second). The following showVideo
example illustrates how to read and play a video file with OpenCV:
//… (omitted for simplicity) int main(int argc, char *argv[]) { Mat frame; // Container for each frame VideoCapture vid(argv[1]); // Open original video file if (!vid.isOpened()) // Check whether the file was opened return -1; int fps = (int)vid.get(CV_CAP_PROP_FPS); namedWindow(argv[1]); // Creates a window while (1) { if (!vid.read(frame)) // Check end of the video file break; imshow(argv[1], frame); // Show current frame on window if (waitKey(1000/fps) >= 0) break; } return 0; }
The code explanation is given as follows:
VideoCapture::VideoCapture(const string& filename)
– This class constructor provides a C++ API to grab a video from the files and cameras. The constructor can have one argument, either a filename or a device index for a camera. In our code example, it is used with a filename obtained from the command-line arguments as follows:VideoCapture vid(argv[1]);
double VideoCapture::get(int propId)
– This method returns the specifiedVideoCapture
property. If a property is not supported by the backend used by theVideoCapture
class, the value returned is0
. In the following example, this method is used to get the frames per second of the video file:int fps = (int)vid.get(CV_CAP_PROP_FPS);
Since the method returns a
double
value, an explicit cast toint
is done.bool VideoCapture::read(Mat& image)
– This method grabs, decodes, and returns a video frame from theVideoCapture
object. The frame is stored in aMat
variable. If it fails (for example, when the end of the file is reached), it returnsfalse
. In the code example, this method is used as follows, also checking the end of file condition:if (!vid.read(frame)) // Check end of the video file break;
In the preceding example, the waitKey
function is used with a computed number of milliseconds (1000/fps
) trying to play the video file at the same rate it was originally recorded. Playing a video at a faster/slower rate (more/less fps) than that will produce a faster/slower playback.