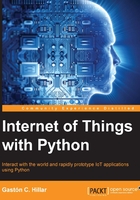
Isolating the pin numbers to improve wirings
Obviously, it is easy to turn on the LED that represents number 1 when it is connected to GPIO pin number 1. In our previous wiring, the LED that represented each number was connected to the same GPIO pin number. The schema was also very easy to understand with the connections where the LED number matched the pin number.
However, the wirings between the board and the breadboard were a bit complicated because the GPIO pins in the board go from 13 down to 1, from left to right. The breadboard has the LEDs in the opposite direction, that is, from 1 to 9, left to right. Thus, the wire that connect the GPIO pin number 1 with LED number 1 has to go from right to left and crosses the other jumper wires. We will change the jumper wires to improve our wiring and then we will make the necessary changes to our object-oriented Python code to isolate the pin numbers and make it possible to have a nicer wiring. Don't forget to shutdown the operating system and unplug the power supply from the board before you make changes to the wirings.
The following diagram shows the components connected to the breadboard and the new wirings from the Intel Galileo Gen 2 board to the breadboard. The Fritzing file for the sample is iot_fritzing_chapter_03_06.fzz
and the following picture is the breadboard view.

Now, whenever we want to turn on LED 1, we must write a high (1) value to GPIO pin number 9, whenever we want to turn on LED 2, we write a high (1) value to GPIO pin number 8, and so on. Because we changed the wirings, the schematic with the electronic components represented as symbols also changed. The following picture shows the new version of the schematic.

The following lines show the new code for the Led
class. The code file for the sample is iot_python_chapter_03_06.py
.
import mraa import time class Led: def __init__(self, pin, position): self.position = position self.gpio = mraa.Gpio(pin) self.gpio.dir(mraa.DIR_OUT) def turn_on(self): self.gpio.write(1) print("I've turned on the LED connected to GPIO Pin #{0}, in position {1}.".format(self.gpio.getPin(), self.position)) def turn_off(self): self.gpio.write(0) print("I've turned off the LED connected to GPIO Pin #{0}, in position {1}.".format(self.gpio.getPin(), self.position))
Now, we have to specify an additional parameter when we create an instance of the Led
class: the position
in the breadboard, that is the LED number in the breadboard. The constructor, that is, the __init__
method, saves the position
value in an attribute with the same name. Both the turn_on
and turn_off
methods use the self.position
attribute value to print a message indicating the position of the LED that has been turned on or off. As the position doesn't match the pin anymore, the message had to be improved to specify the position.
The following lines show the code for the new version of the NumberInLeds
class. The code file for the sample is iot_python_chapter_03_06.py
.
class NumberInLeds: def __init__(self): self.leds = [] for i in range(9, 0, -1): led = Led(i, 10 - i) self.leds.append(led) def print_number(self, number): print("==== Turning on {0} LEDs ====".format(number)) for j in range(0, number): self.leds[j].turn_on() for k in range(number, 9): self.leds[k].turn_off()
It was necessary to make changes to the highlighted lines in the constructor, that is, the __init__
method. The for
loop that creates the nine instances of the Led
class now starts with i
equal to 9 and its last itearation will be with i
equal to 1. We pass i
as an argument for the pin
parameter and 10 – i
as an argument for the position parameter
. This way, the first Led
instance in the self.leds
list will be the one with pin equal to 9 and position equal to 1.
The code that uses the new version of the NumberInLeds
class to count from 0 to 9 with the LEDs is the same than the previous code. The code file for the sample is iot_python_chapter_03_06.py
.
if __name__ == "__main__": print ("Mraa library version: {0}".format(mraa.getVersion())) print ("Mraa detected platform name: {0}".format(mraa.getPlatformName())) number_in_leds = NumberInLeds() # Count from 0 to 9 for i in range(0, 10): number_in_leds.print_number(i) time.sleep(3)
We just needed to make a few changes in the class that encapsulates a LED (Led
) and in the class that encapsulates a number represented with LEDs (NumberInLeds
). The following picture shows the 9 LEDs turned on in the breadboard with the new wirings connected between the breadboard and the board that is running the new Python code running.

We can easily build an API and provide a REST API to allow any client that has connection to the board to be able to print numbers through HTTP. Our REST API just needs to create an instance of the NumberInLeds
class and call the print_number
method with the specified number to be printed with LEDs. We will build this REST API in the next chapter.