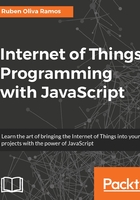
Configuring the one-wire protocol
Open a terminal in the Raspberry Pi, and type the following:
sudo nano /boot/config.txt
You should type the following line at the bottom of the page to configure the protocol and define the pin where the one-wire protocol will communicate:
dtoverlay=w1-gpio
The next step is to reboot the Raspberry Pi. After a few minutes, open the terminal and type the following lines:
sudo modprobew1-gpio sudo modprobe w1-therm
Enter the folder and select the device that will be configured:
cd /sys/bus/w1/devices ls
Select the device that will be set up. Change xxxx
to the serial number of the device that will set up in the protocol:
cd 28-xxxx cat w1_slave
You will see the following:

After that, you will see one line which says Yes if it appears that the temperature reading is done like this: t=29.562.
Software configuration
Let's now look at the code to display the temperature in degrees Celsius and Fahrenheit every second on the screen.
Here we import the libraries used in the program:
import os1 import glob1 import time1
Here we define the devices configured in the protocol:
os1.system('modprobew1-gpio') os1.system('modprobew1-therm1')
Here we define the folders where the devices are configured:
directory = '/sys/bus/w1/devices/' device_folder1 = glob1.glob(directory + '28*')[0] device_file1 = device_folder1 + '/w1_slave'
Then we define the functions to read temperature
and configure the sensor:
defread_temp(): f = open(device_file1, 'r') readings = f.readlines() f.close() return readings
Read the temperature with the function:
defread_temp(): readings = read_temp()
In this function, we compare when it received the message YES
and get the t=
character. We also get the value of the temperature:
while readings[0].strip()[-3:] != 'YES': time1.sleep(0.2) readings = read_temp() equals = lines[1].find('t=')
Then we calculate the temperature, temp
in C
and F
, and return the values:
if equals != -1: temp = readings[1][equals pos+2:] tempc = float(temp) / 1000.0 tempf = temp * 9.0 / 5.0 + 32.0 returntempc, tempf
It repeats the cycle every second:
while True: print(temp()) time1.sleep(1)
Displaying the readings on the screen
Now we need to execute thermometer.py
. To show the results of the scripts made in Python, open your PuTTY terminal, and type the following command:
sudo python thermometer.py
The command means that, when we run the thermometer file, if everything is running perfectly, we will see the following results:
