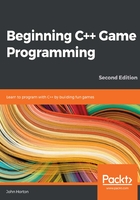
Preparing the player (and other sprites)
Let's add the code for the player's sprite as well as a few more sprites and textures at the same time. The following, quite large, block of code also adds a gravestone sprite for when the player gets squashed, an axe sprite to chop with, and a log sprite that can whiz away each time the player chops.
Notice that, after the spritePlayer object, we declare a side variable, playerSide, to keep track of where the player is currently standing. Furthermore, we add some extra variables for the spriteLog object, including logSpeedX, logSpeedY, and logActive, to store how fast the log will move and whether it is currently moving. The spriteAxe also has two related float constant variables to remember where the ideal pixel position is on both the left and the right.
Add the following block of code just before the while(window.isOpen()) code, like we have done so often before. Note that all of the code in the following block is new, not just the highlighted code. I haven't provided any extra context for this block of code as the while(window.isOpen()) should be easy to identify. The highlighted code is the code we have just discussed.
Add the entirety of the following code, just before the while(window.isOpen()) line, and make a mental note of the highlighted lines we briefly discussed. It will make the rest of this chapter's code easier to understand:
// Prepare the player
Texture texturePlayer;
texturePlayer.loadFromFile("graphics/player.png");
Sprite spritePlayer;
spritePlayer.setTexture(texturePlayer);
spritePlayer.setPosition(580, 720);
// The player starts on the left
side playerSide = side::LEFT;
// Prepare the gravestone
Texture textureRIP;
textureRIP.loadFromFile("graphics/rip.png");
Sprite spriteRIP;
spriteRIP.setTexture(textureRIP);
spriteRIP.setPosition(600, 860);
// Prepare the axe
Texture textureAxe;
textureAxe.loadFromFile("graphics/axe.png");
Sprite spriteAxe;
spriteAxe.setTexture(textureAxe);
spriteAxe.setPosition(700, 830);
// Line the axe up with the tree
const float AXE_POSITION_LEFT = 700;
const float AXE_POSITION_RIGHT = 1075;
// Prepare the flying log
Texture textureLog;
textureLog.loadFromFile("graphics/log.png");
Sprite spriteLog;
spriteLog.setTexture(textureLog);
spriteLog.setPosition(810, 720);
// Some other useful log related variables
bool logActive = false;
float logSpeedX = 1000;
float logSpeedY = -1500;
In the preceding code, we added quite a few new variables. They are hard to explain in full until we get to where we actually use them, but here is an overview of what they will be used for. There is a variable of the side enumeration type called playerSide that is initialized to left. This will track which side of the tree the player is on.
There are two const float values that determine the horizontal position the axe will be drawn at, depending on whether the player is on the left-or right-hand side of the tree.
There are also three variables to help to keep control of the log as it is chopped and flies off of the tree, bool to determine whether the log is in motion (logActive) and two float values to hold the horizontal and vertical speeds of the log.
Now, we can draw all of our new sprites.