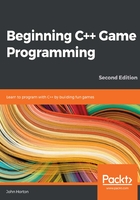
C++ Strings
In the previous chapter, we briefly mentioned Strings and we learned that a String can hold alphanumeric data – anything from a single character to a whole book. We didn't look at declaring, initializing, or manipulating Strings, so let's do that now.
Declaring Strings
Declaring a String variable is simple. It is the same process that we used for other variables in the previous chapter: we state the type, followed by the name:
String levelName;
String playerName;
Once we have declared a String, we can assign a value to it.
Assigning a value to a String
To assign a value to a String, just like regular variables, we simply put the name, followed by the assignment operator, and then the value:
levelName = "DastardlyCave";
playerName = "John Carmack";
Note that the values need to be enclosed in quotation marks. Just like regular variables, we can also declare and assign values in a single line:
String score = "Score = 0";
String message = "GAME OVER!!";
In the next section, we will see how we can change the values of our String variables.
Manipulating Strings
We can use the #include <sstream> directive to give us some extra manipulation options for our Strings. The sstream class allows us to "add" some Strings together. When we add Strings together, this is known as concatenation:
String part1 = "Hello ";
String part2 = "World";
sstream ss;
ss<< part1 << part2;
// ss now holds "Hello World"
In addition to this, by using sstream objects a String variable can even be concatenated with a variable of a different type. The following code starts to reveal how Strings might be useful to us:
String scoreText = "Score = ";
int score = 0;
// Later in the code
score ++;
sstream ss;
ss<<scoreText<< score;
// ss now holds "Score = 1"
In the preceding code, ss is used to join the content of scoreText with the value from score. Note that although score holds an int value, the final value held by ss is still a String that holds an equivalent value; in this case, "1".
Tip
The << operator is one of the bitwise operators. C++, however, allows you to write your own classes and override what a specific operator does within the context of your class. The sstream class has done this to make the << operator work the way it does. The complexity is hidden in the class. We can use its functionality without worrying about how it works. If you are feeling adventurous, you can read about operator overloading at http://www.tutorialspoint.com/cplusplus/cpp_overloading.htm. You don't need any more information in order to continue with the project.
Now that we know the basics of C++ Strings and how we can use sstream, we will look at how we can use some SFML classes to display them on the screen.