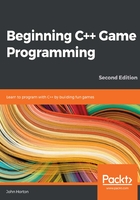
C++ variables
Variables are the way that our C++ games store and manipulate values/data. If we want to know how much health the player has, we need a variable. Perhaps you want to know how many zombies are left in the current wave. That is a variable as well. If you need to remember the name of the player who got a high score, you guessed it—we need a variable for that. Is the game over or still playing? Yes, that's a variable too.
Variables are named identifiers for locations in the memory of the PC. The memory of the PC is where computer programs are stored as they are being executed. So, we might name a variable numberOfZombies and that variable could refer to a place in memory that stores a value to represent the number of zombies that are left in the current wave.
The way that computer systems address locations in memory is complex. Programming languages use variables to give us a human-friendly way to manage our data in that memory.
The small amount we have just mentioned about variables implies that there must be different types of variable.
Types of variables
There is a wide variety of C++ variable types (see the next tip about variables in a couple of pages). It would easily be possible to spend an entire chapter discussing them. What follows is a table of the most commonly used types of variable in this book. Then, in the next section, we will look at how to use each of these variable types:

The compiler must be told what type of variable it is so that it can allocate the right amount of memory for it. It is good practice to use the best and most appropriate type for each variable you use. In practice, however, you will often get away with promoting a variable. Perhaps you need a floating-point number with just five significant digits? The compiler won't complain if you store it as a double. However, if you tried to store a float or a double in an int, it will change/cast the value to fit the int. As we progress through this book, I will make it plain what the best variable type to use in each case is, and we will even see a few instances where we deliberately convert/cast between variable types.
A few extra details worth noticing in the preceding table include the f postfix next to all of the float values. This f postfix tells the compiler that the value is a float type, not double. A floating-point value without the f prefix is assumed to be double. See the next tip about variables for more about this.
As we mentioned previously, there are many more types. If you want to find out more about types, see the next tip about variables.
User-defined types
User-defined types are way more advanced than the types we have just seen. When we talk about user-defined types in C++, we are usually talking about classes. We briefly talked about classes and their related objects in the previous chapter. We would write code in a separate file, sometimes two. We are then able to declare, initialize, and use them. We will leave how we define/create our own types until Chapter 6, Object-Oriented Programming – Starting the Pong Game.
Declaring and initializing variables
So far, we know that variables are for storing the data/values that our games need in order to work. For example, a variable would represent the number of lives a player has or the player's name. We also know that there is a wide selection of different types of values that these variables can represent, such as int, float, bool, and so on. Of course, what we haven't seen yet is how we would actually go about using a variable.
There are two stages when it comes to creating and preparing a new variable. These stages are called declaration and initialization.
Declaring variables
We can declare variables in C++ like this:
// What is the player's score?
int playerScore;
// What is the player's first initial
char playerInitial;
// What is the value of pi
float valuePi;
// Is the player alive or dead?
bool isAlive;
Once we have written the code to declare a variable, it exists and is ready to be used in our code. However, we will usually want to give the variable an appropriate value, which is where initialization comes in.
Initializing variables
Now that we have declared the variables with meaningful names, we can initialize those same variables with appropriate values, like this:
playerScore = 0;
playerInitial = 'J';
valuePi = 3.141f;
isAlive = true;
At this point, the variable exists and holds a specific value. Soon, we will see how we can change, test, and respond to these values. Next, we will see that we can combine declaring and initializing into one step.
Declaring and initializing in one step
When it suits us, we can combine the declaration and initialization steps into one. Sometimes, we know what value a variable must start the program with, and declaring and initializing in one step is appropriate. Often, we won't, and we will first declare the variable and then initialize it later in the program, like so:
int playerScore = 0;
char playerInitial = 'J';
float valuePi = 3.141f;
bool isAlive = true;
Variables tip
As promised, here is the tip on variables. If you want to see a complete list of C++ types, then check out this web page: http://www.tutorialspoint.com/cplusplus/cpp_data_types.htm. If you want a deeper discussion on float, double, and the f postfix, then read this: http://www.cplusplus.com/forum/beginner/24483/. Finally, if you want to know the ins and outs of the ASCII character codes, then there is some more information here: http://www.cplusplus.com/doc/ascii/. Note that these links are for the extra curious reader and we have already discussed enough in order to proceed.
Constants
Sometimes, we need to make sure that a value can never be changed. To achieve this, we can declare and initialize a constant using the const keyword:
const float PI = 3.141f;
const int PLANETS_IN_SOLAR_SYSTEM = 8;
const int NUMBER_OF_ENEMIES = 2000;
It is convention to declare constants in all uppercase. The values of the preceding constants can never be altered. We will see some constants in action in Chapter 4, Loops, Arrays, Switches, Enumerations, and Functions – Implementing Game Mechanics.
Declaring and initializing user-defined types
We have already seen examples of how we can declare and initialize some SFML defined types. It is because of the way that we can create/define these types (classes) so flexibly that the way we declare and initialize them is also so varied. Here are a couple of reminders for declaring and initializing user-defined types from the previous chapter.
Create an object of the VideoMode type called vm and initialize it with two int values, 1920 and 1080:
// Create a video mode object
VideoMode vm(1920, 1080);
Create an object of the Texture type called textureBackground, but don't do any initialization:
// Create a texture to hold a graphic on the GPU
Texture textureBackground;
Note that it is possible (in fact, very likely) that even though we are not suggesting any specific values with which to initialize textureBackground, some setup of variables may take place internally. Whether or not an object needs/has the option of giving initialization values at this point is entirely dependent on how the class is coded and is almost infinitely flexible. This further suggests that, when we get to write our own classes, there will be some complexity. Fortunately, this also means we will have significant power to design our types/classes to be just what we need to make our games! Add this huge flexibility to the power of the SFML designed classes and the potential for our games is almost limitless.
We will see a few more user created types/classes provided by SFML in this chapter too, and loads more throughout this book.
We have now seen that a variable is a named location in the computer's memory and that a variable can be a simple integer through to a more powerful object. Now that we know we can initialize these variables, we will look at how we can manipulate the values they hold.