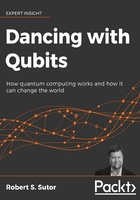
if statements and conditions
Now I would like to introduce you to a very useful tool in programming – if
conditions!
They are widely used to check whether a statement is true or not. If the given statement is true, then some instructions for our code are followed.
I'll present this subject to you with some simple code that will tell us whether a number is positive, negative, or equal to 0. The code's very short, so I'll show you all of it at once:
a = 5
if a > 0:
print('a is greater than 0')
elif a == 0:
print('a is equal to 0')
else:
print('a is lower than 0')
In the first line, we introduce a new variable called a
and we give it a value of 5
. This is the variable whose value we are going to check.
In the next line we check if this variable is greater than 0
. We do this by using an if
condition. If a
is greater than 0
, then we follow the instructions written in the indented block; in this case, it is only displaying the message a is greater than 0
.
Then, if the first condition fails, that is, if a
is lower than or equal to 0
, we go to the next condition, which is introduced with elif
(which is short for else if
). This statement will check whether a
is equal to zero or not. If it is, we follow the indented instruction, which will display a message displaying: a is equal to 0
.
The final condition is introduced via else
. Instructions included in an else
condition will always be followed when all other conditions fail. In this case, failing both conditions would mean that a
< 0
, and therefore we would display a is lower than 0
.
It's easy to predict what our code will return. It will be the first instruction, print('a is greater than 0')
. And, in fact, once you run this code, this is what you will get:
a is greater than 0
In brief, if
is used to introduce statement checking and the first condition, elif
is used to check as many further conditions as we want, and else
is a true statement when all other statements fail.
It's also important to know that once one condition is true, no other conditions are checked. So, in this case, once we enter the first condition and we see that it is true, we no longer check other statements. If you would like to check other conditions, you would need to replace the elif
and else
statements with new if
statements. A new if
always checks a new condition; therefore, a condition included in an if
is always checked.
Exercise
Build a condition that will check if a number is divisible by 3 or not.
Hint: You can use a mathematical expression called modulo, which when used, returns the remainder from the division between two numbers. In Python, modulo is represented by %
. For example:
5 % 3 = 2
71 % 5 = 1
The solution is provided in the Chapter 03/If Statements/homework.py
file on the GitHub page.