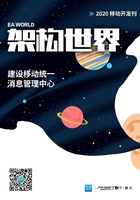
上QQ阅读APP看书,第一时间看更新
三、如何实现二维码管理系统
二维码的生成
推荐在二维码标准选择的时候使用应用最为广泛的
码,后续代码示例中也会以此为基准。目前开源的二维码生成工具包有很多,比如
、 、 、 等。这里选用一个比较常用的由 开发维护的 ,具体的代码可以参考 代码测试包中的 类来辅助开发,可以将该类直接拷贝到源码中使用。= . ();
= . ();
= ( , , . _ _ );
( = ; < ; ++) {
( = ; < ; ++) {
. ( , ,( . ( , ) ? : ));
}
}
;
}
( , , ) {
= ( );
= ();
= . ( );
(! . ( , , )) {
(" " + + " " + );
}
}
对于部分业务系统在生成二维码的时候可能存在需要在二维码图片中心添加
图片的业务需求,这里贴出在二维码中添加 的示例:
( , ) {
= . ();
= . ();
= . ();
= . ( ( ));
. ( , / * , / * , / , / , );
= ( , . _ , . _ );
. ( );
. = . ( / * , / * ,
/ , / , , );
. ( . );
. ( );
= ( , . _ , . _ );
. ( );
. = . ( / * + ,
/ * + , / - , / - , , );
. ( ( , , ));
. ( );
. ();
. () ;
;
}
二维码内容的加解密
二维码内容加密主要分为两部分,其一是生成用于完整性校验字段的哈希算法,其二是为了明文加密的对称加密。
常用于完整性检验的哈希算法有很多种,如
( 公司)的消息摘要算法 系列,这其中 因为兼具快速和安全两大特点被广泛采。同样的哈希算法还有美国国家标准技术研究院( )制定的安全哈希算法 ( )系列算法,国家密码管理局发布的杂凑算法标准 等。这里使用 作为代码范例:( ) {
= ;
[] = . ();
{
= . (" ");
}
( ) {. ();
}
. ( , , . );
= ( , . ());
. ( );
}
在二维码密文解析的时候可使用相同方法取原文的
值进行检验。二维码明文加密采用对称加密算法,对称密钥由二维码管理系统保管,常见的对称算法有
算法、 算法、 算法、 算法等,这里以 算法为例。加密:
( , ) {
{
= . ( _ _ );// 创建密码器
[] = . (" - ");
. ( . _ , ( ));// 初始化为加密模式的密码器
[] = . ( );// 加密
. ( );//通过 转码返回
}
( ) {. ( . . ()). ( . , , );
}
;
}
解密:
( , ) {
{
//实例化
= . ( _ _ );
//使用密钥初始化,设置为解密模式
. ( . _ , ( ));
//执行操作
[] = . ( . ( ));
( , " - ");
}
( ) {. ( . . ()). ( . , , );
}
;
}
生成密钥:
( ) {
//返回生成指定算法密钥生成器的
对象= ;
{
= . ( _ );
//
要求密钥长度为. ( , ( . ()));
//生成一个密钥
= . ();
( . (), _ );// 转换为 专用密钥
}
( ) {. ( . . ()). ( . , , );
}
;
}
这里简要说明了加解密的相关示例,网上也有相关的开源示例,对加解密有兴趣的话可以自行深入研究。
推荐阅读
浅析 端的消息推送原理

关于作者:风行云,现任普元信息移动团队开发工程师,毕业于山东大学(威海),主攻移动原生开发、 开发、 开发。先后参与中国邮政集团移动平台、国家开发银行移动应用平台等项目的开发工作。