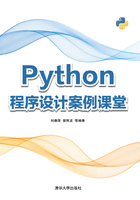
6.5 类的内置方法
类本身有许多内置方法,这些内置方法的开头与结尾都是双底线字符。具体介绍如下。
(1)__init__(self):这是类的构造方法,当创建一个类的实例时,就会调用此方法。下列案例设置类的构造方法是打印类实例本身:
>>>class myClass: def __init__(self): print (self) >>>x = myClass() <__main__.myClass object at 0x02D91630>
(2)__str__(self):此方法被str()内置函数与print函数调用,用来设置对象以字符串类型出现时如何显示,__str__()函数的返回值是一个字符串对象。下列案例的print函数会打印出类实例的name属性:
>>> class myClass: def __init__(self, arg): self.name = arg def __str__(self): return self.name >>>x = myClass("张三丰") >>>print (x) 张三丰
(3)__repr__(self):此方法被repr()内置函数调用,此函数可以让对象以可读的形式出现。下列案例在提示符号后列出类实例变量的名称时,即打印出类实例变量的name属性:
>>> class myClass: def __init__(self, arg): self.name = arg def __repr__(self): return self.name >>> x = myClass("小明") >>> x '小明’
(4)__getattr__(self, name):此方法用于读取或是修改不存在的成员属性的时候。下列案例在读取类实例的属性时,返回属性值:
>>> class myClass: def __init__(self, arg): self.name = arg def __getattr__(self, name): return name >>> x = myClass("张晓明") >>> x.s 's'
(5)__setattr__(self, name, value):此方法用于设置类属性的值。下列案例在设置类实例的name属性时,在属性值之后加上“is male”字符串:
>>> class myClass: def __init__(self, arg): self.name = arg def __setattr__(self, name, value): self.__dict__[name] = value + " is male" >>> x = myClass("张小明") >>> x.name = "张小华" >>> x.name '张小华is male'
(6)__delattr__(self, name):此方法用于删除类的属性。下列案例在使用delattr语句删除name属性时,显示“你不能删除此类的属性”字符串:
>>> class myClass: def __init__(self, arg): self.name = arg def __delattr__(self, name): print ("你不能删除此类的属性") >>> x = myClass("Andre") >>> del x.name 你不能删除此类的属性
(7)__del__(self):此方法用于删除类对象。下列案例在使用del语句删除类实例时,显示“你不能删除此类的对象”字符串:
>>class myClass: def __init__(self, arg): self.name = arg def __del__(self): print ("你不能删除此类的对象") >>>x = myClass("Andre") >>> del x 你不能删除此类的对象
(8)__hash__(self):此方法用来产生32位的哈希索引值。例如:
>>> class hashNumber: def __init__(self, arg): self.value = arg def __hash__(self): return self.value >>> x = hashNumber(10000) >>> hash(x) 10000
(9)__nonzero__(self):此方法用于测试布尔值时,返回0或是1。例如
>>> class nonzeroNumber: def __init__(self, arg): self.value = arg def __nonzero__(self): if self.value: return 1 else: return 0 >>> x = nonzeroNumber([1,2,3]) >>> x.__nonzero__() 1 >>> y = nonzeroNumber("") >>> y.__nonzero__() 0
(10)__call__(self):若类内包含此方法,是可以被调用的。下列案例调用x类实例时,返回原来name属性值与调用时的参数相加的结果:
>>> class addNumber: def __init__(self, arg): self.value = arg def __call__(self, other): return self.value + other >>> x = addNumber(10) >>> x(40) 50
(11)__getitem__(self, index):此方法支持列表对象的索引,返回self[index]值。下列案例显示列表对象的元素时,将元素值设置成索引值加1:
>>> class Seq: def __getitem__(self, index): return index + 1 >>> s = Seq() >>> for i in range(8): print (s[i]) 1 2 3 4 5 6 7 8
(12)__len__(self):此方法用在len()内置函数显示类实例变量的长度时。下列案例返回类实例x的name属性的长度值:
>>> class myClass: def __init__(self, arg): self.name = arg def __len__(self): return len(self.name) >>> x = myClass("Hello Python") >>> len(x) 12
(13)__add__(self, other):此方法用于计算self + other的值。下列案例返回类的两个实例x与y相加的结果:
>>> class addNumber: def __init__(self, x, y): self.x = x self.y = y def __add__(self, other): return (self.x + other.x, self.y + other.y) >>> x = addNumber(2, 4) >>> y = addNumber(7, 3) >>> print (x + y) (9, 7)
(14)__iadd__(self, other):此方法用于计算self += other的值。下列案例将类的两个实例x与y相加的结果设置给类实例x:
>>> class iaddNumber: def __init__(self, arg): self.value = arg def __iadd__(self, other): return self.value + other.value >>> x = iaddNumber(12) >>> y = iaddNumber(10) >>> x += y >>> x 22
(15)__sub__(self, other):此方法用于计算self - other的值。下列案例返回类的两个实例x与y相减的结果:
>>> class subNumber: def __init__(self, value): self.value = value def __sub__(self, other): return (self.value - other.value) >>> x = subNumber(100) >>> y = subNumber(30) >>>print (x - y) 70
(16)__isub__(self, other):此方法用于计算self -= other的值。下列案例将类的两个实例x与y相减的结果设置给类实例x:
>>>class isubNumber: def __init__(self, arg): self.value = arg def __isub__(self, other): return self.value - other.value >>> x = isubNumber(12) >>> y = isubNumber(10) >>> x -= y >>> x 2
(17)__mul__(self, other):此方法用于计算self * other的值。下列案例返回类的两个实例x与y相乘的结果:
>>>class mulNumber: def __init__(self, value): self.value = value def __mul__(self, other): return (self.value * other.value) >>> x = mulNumber(12) >>> y = mulNumber(4) >>> print (x * y) 48
(18)__imul__(self, other):此方法计算self *= other的值。下列案例将类的两个实例x与y相乘的结果设置给类实例x:
>>>class imulNumber: def __init__(self, arg): self.value = arg def __imul__(self, other): return self.value * other.value >>> x = imulNumber(12) >>> y = imulNumber(10) >>> x *= y >>> x 120
(19)__mod__(self, other):此方法用于计算self % other的值。下列案例返回类的两个实例x与y相除的余数:
>>> class modNumber: def __init__(self, value): self.value = value def __mod__(self, other): return (self.value % other.value) >>> x = modNumber(10) >>> y = modNumber(3) >>> print (x % y) 1
(20)__imod__(self, other):此方法用于计算self %= other的值。下列案例将类的两个实例x与y相除的余数设置给类实例x:
>>> class imodNumber: def __init__(self, arg): self.value = arg def __imod__(self, other): return self.value % other.value >>> x = imodNumber(12) >>> y = imodNumber(10) >>> x %= y >>> x 2
(21)__neg__(self):此方法用于计算-self的结果。下列案例返回类实例x之前加一个符号(-)的结果:
>>> class negNumber: def __init__(self, value): self.value = value def __neg__(self): return -self.value >>> x = negNumber(-10) >>> print (-x) 10
(22)__pos__(self):此方法用于计算+self的结果。下列案例返回类实例x之前加一个符号(+)的结果:
>>> class posNumber: def __init__(self, value): self.value = value def __pos__(self): return self.value >>> x = posNumber(-10) >>> print (+x) -10