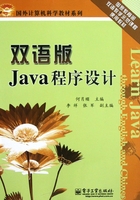
1.4 Main Features of Java Programming Language
1.4.1 Portability
Most modern programming languages are designed to be (relatively) easy for people to write and to understand. These languages that were designed for people to read are called high-level languages. The language that the computer can directly understand is called machine language. Machine language or any language similar to machine language is called a low-level language. A program written in a high-level language, like Java, must be translated into a program in machine language before the program can be run. The program that does the translating (or at least most of the translating) is called a compiler and the translation process is called compiling. The Java compiler does not translate your program into the machine language for your particular computer. Instead, it translates your Java program into a language called byte-code(字节码). Byte-code is not the machine language for any particular computer. Byte-code is the machine language for a fictitious computer called the Java Virtual Machine(Java虚拟机).
Java作为一种高级编程语言,具有很强的移植性,通过Java虚拟机可将字节码运行在不同的机器平台上。
The reason is: only the JVM needs to be implemented for each platform. Once the run-time package exists for a given system, any Java program can run on it. Remember, although the details of the JVM will differ from platform to platform, all interpret the same Java bytecode. If a Java program were compiled to native code, then different versions of the same program would have to exist for each type of CPU connected to the Internet. This is, of course, not a feasible solution. Thus, the interpretation of bytecode is the easiest way to create truly portable programs. The fact that a Java program is interpreted also helps to make it secure. Because the execution of every Java program is under the control of the JVM, the JVM can contain the program and prevent it from generating side effects outside of the system. As you will see, safety is also enhanced by certain restrictions that exist in the Java language.
JVM是Java跨平台的基础,Java源程序被编译成字节码,然后交给JVM解释执行。这样Java就不需要知道自己是在何种硬件平台下运行的。
When a program is interpreted, it generally runs substantially slower than it would run if compiled to executable code. However, with Java, the differential between the two is not so great. The use of bytecode enables the Java run-time system to execute programs much faster than you might expect. Although Java was designed for interpretation, there is technically nothing about Java that prevents on-the-fly compilation of bytecode into native code. Along these lines, Sun supplies its Just In Time (JIT) compiler for bytecode, which is included in the Java 2 release. When the JIT compiler is part of the JVM, it compiles bytecode into executable code in real time, on a piece-by-piece, demand basis. It is important to understand that it is not possible to compile an entire Java program into executable code all at once, because Java performs various run-time checks that can be done only at run time. Instead, the JIT compiles code, as it is needed, during execution. However, the just-in-time approach still yields a significant performance boost. Even when dynamic compilation is applied to bytecode, the portability and safety features still apply, because the run-time system (which performs the compilation) still is in charge of the execution environment. Whether your Java program is actually interpreted in the traditional way or compiled on the fly, its functionality is the same.
JIT是JVM的一部分,它可以按要求即时地将字节码逐一地编译为可执行的代码。
1.4.2 Simple
We wanted to build a system that could be programmed easily without a lot of esoteric training and which leveraged today’s standard practice. Most programmers working these days use C, and most programmers doing object-oriented programming use C++. So even though we found that C++ was unsuitable, we designed Java as closely to C++ as possible in order to make the system more comprehensible.
Java omits many rarely used, poorly understood, confusing features of C++ that in our experience bring more grief than benefit. These omitted features primarily consist of operator overloading(although the Java language does have method overloading), multiple inheritance, and extensive automatic coercions.
We added automatic garbage collection, thereby simplifying the task of Java programming but making the system somewhat more complicated. A common source of complexity in many C and C++ applications is storage management: the allocation and freeing of memory. By virtue of having automatic garbage collection (periodic freeing of memory not being referenced) the Java language not only makes the programming task easier, it also dramatically cuts down on bugs.
Java是一种非常简单的编程语言,优化了C++语言中一些令人难以理解的特性,增加了诸如垃圾自动回收机制的内容,方便了初学者的学习和使用。
1.4.3 Robust
The multi-plat formed environment of the Web places extraordinary demands on a program, because the program must execute reliably in a variety of systems. Thus, the ability to create robust programs was given a high priority in the design of Java. To gain reliability, Java restricts you in a few key areas, to force you to find your mistakes early in program development. At the same time, Java frees you from having to worry about many of the most common causes of programming errors. Because Java is a strictly typed language, it checks your code at compile time. However, it also checks your code at run time. In fact, many hard-to-track-down bugs that often turn up in hard-to-reproduce run-time situations are simply impossible to create in Java. Knowing that what you have written will behave in a predictable way under diverse conditions is a key feature of Java. To better understand how Java is robust, consider two of the main reasons for program failure: memory management mistakes and mishandled exceptional conditions (that is, run-time errors).
在多平台环境中,健壮性是一个程序的重要指标,Java有着严格的语法规范,它可以在编译时和运行时检查所编写的代码。Java主要考虑了会导致程序运行失败的两个方面:内存管理和不可处理的异常。
One of the advantages of a strongly typed language (like C++) is that it allows extensive compile-time checking so bugs can be found early. Unfortunately, C++ inherits a number of loopholes in compile-time(编译期间) checking from C, which is relatively lax (particularly method/procedure declarations). In Java, we require declarations and do not support C-style implicit declarations. The linker understands the type system and repeats many of the type checks done by the compiler to guard against version mismatch problems. The single biggest difference between Java and C/C++ is that Java has a pointer model that eliminates the possibility of overwriting memory and corrupting data. Instead of pointer arithmetic, Java has true arrays. This allows subscript checking to be performed. In addition, it is not possible to turn an arbitrary integer into a pointer by casting.
Java程序在一些关键的地方做了强制性限制,这样使开发者很容易发现自己所犯的错误。同样,Java也解除了很多开发者认为可能会引起程序错误的内容。
1.4.4 Multithread
Java was designed to meet the real-world requirement of creating interactive, networked programs. To accomplish this, Java supports multithread programming, which allows you to write programs that do many things simultaneously(同时地). The Java run-time system comes with an elegant yet sophisticated solution for multiprocessor Synchronization(多处理器同步) that enables you to construct smoothly running interactive systems. Java’s easy-to-use approach to multithread allows you to think about the specific behavior of your program, not the multitasking subsystem.
Java提供易使用的多线程允许开发者考虑程序中所需要的特定行为。
1.4.5 Architecture-Neutral
A central issue for the Java designers was that of code longevity and portability. One of the main problems facing programmers is that no guarantee exists that if you write a program today, it will run tomorrow—even on the same machine. Operating system upgrades, processor upgrades, and changes in core system resources can all combine to make a program malfunction. The Java designers made several hard decisions in the Java language and the Java Virtual Machine in an attempt to alter this situation. Their goal was “write once, run anywhere, any time, forever.” To a great extent, this goal was accomplished.
体系结构中性可以保证Java程序能在不同平台上运行,实现“一次编写,处处运行”的目标。
1.4.6 Interpreted and High Performance
As described earlier, Java enables the creation of cross-platform programs by compiling into an intermediate representation called Java byte code. This code can be interpreted on any system that provides a Java Virtual Machine. Most previous attempts at cross-platform solutions have done so at the expense of performance. Other interpreted systems, such as BASIC, TCL and PERL, suffer from almost insurmountable performance deficits. Java, however, was designed to perform well on very low-power CPUs. As explained earlier,the Java byte code was carefully designed so that it would be easy to translate directly into native machine code for very high performance by using a just-in-time compiler.
Java程序占有少量的CPU资源,其字节码能够很容易被直接翻译成机器代码。
1.4.7 Distributed
Java is designed for the distributed environment of the Internet, because it handles TCP/IP protocols. In fact, accessing a resource using a URL is not much different from accessing a file. The original version of Java included features for intra-address-space messaging. This allowed objects on two different computers to execute procedures remotely. Java revived these interfaces in a package called Remote Method Invocation. This feature brings an unparalleled level of abstraction to client/server programming.
Java是一种能够在Internet的分布式环境下运行的语言,通过远程方法调用的接口包来实现远程访问。
1.4.8 Dynamic
Java programs carry with them substantial amounts of run-time type information that is used to verify and resolve accesses to objects at run time. This makes it possible to dynamically link code in a safe and expedient manner. This is crucial to the robustness of the applet environment, in which small fragments of byte code may be dynamically updated on a running system.
Java程序可以在运行时动态地加载所需要的对象,这对于Applet小程序的运行非常重要。
1.4.9 Security
The fundamental problem in the internet associated with Java is security. Java’s security model is one of the language’s key architectural features that make it an appropriate technology for networked environments. Security is important because networks provide a potential avenue of attack to any computer hooked to them. This concern becomes especially strong in an environment in which software is downloaded across the network and executed locally, as is done with Java applets, for example. Because the class files for an applet are automatically downloaded when a user goes to the containing Web page in a browser, it is likely that a user will encounter applets from untrusted sources. Without any security, this would be a convenient way to spread viruses. Thus, Java’s security mechanisms help make Java suitable for networks because they establish a needed trust in the safety of network-mobile code.
Java安全模型是Java语言体系结构的关键性特性。它使得Java语言能够适应网络环境。因为这种安全措施在网络代码上建立了一种信任感。