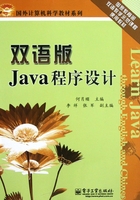
3.10 Variable
Variables are used in a Java program to contain data that changes during the execution of the program.
3.10.1 Declaring a Variable
To use variables, you must notify the compiler of the name and the type of the variable (declare the variable).
The syntax for declaring a variable in Java is to precede the name of the variable with the name of the type of the variable as shown below. It is also possible (but not required) to initialize a variable in Java when it is declared as shown.
Java中声明变量:在变量名称前加变量的类型名称。Java在声明变量时也可初始化(但不是必需的)变量。
int ch1, ch2 = '0';
This statement declares two variables of type int, initializing one of them to the value of the zero character (0).
3.10.2 Difference between Zero and ‘0’-Unicode Characters
The value of the zero character is not the same as the numeric value of zero, but hopefully you already knew that. As an aside, characters in Java are 16-bit entities called Unicode characters instead of 8-bit entities as is the case with many programming languages. The purpose is to provide many more possible characters including characters used in alphabets(字母表)other than the one used in the United States..
3.10.3 Initialization of the Variable
Initialization of the variable named ch2 in this case was necessary to prevent a compiler error. Without initialization of this variable, the compiler recognized and balked at the possibility that an attempt might be made to execute the following statement with a variable named ch2 that had not been initialized.
3.10.4 Error Checking by the Compiler
The strong error-checking capability of the Java compiler refused to compile this program until that possibility was eliminated by initializing the variable.
System.out.println("The char before the # was" + (char)ch2);
Java编译器错误检测功能会拒绝编译一个没有初始化变量的程序。
3.10.5 Using the Cast Operator
You should also note that the variable ch2 is being cast as a char in the above statement. Recall that ch2 is a variable of type int, but we want to display the character that the numeric value represents. Therefore, we must cast it (purposely change its type for the evaluation of the expression). Otherwise, we would not see a character on the screen. Rather, we would see the numeric value that represents that character.
3.10.6 Why Declare the Variables as Type Int?
It was necessary to declare these variables as type int because the highlighted portion of the following statement returns an int.
while((ch1 = System.in.read()) != '#') ch2 = ch1;
Java provides very strict type checking and generally refuses to compile statements with type mismatches(错配).
3.10.7 Shortcut Declaring Variables of the Same Type
There is a short cut which can be taken when declaring many variables of the same type, you can put them all on the same line, after the data type, separating the names with commas.
声明同一类型的多个变量,可以将它们放置在数据类型后的同一行,并用逗号分开。
//declaring variables of the same type on the same line int counter, age, number;
3.10.8 Assigning Values to Variables
Once a variable has been declared then assigning a value to it takes the following syntax:
variable_name = value;
But the value must be of the same type, see the examples below:
//声明一个变量 int counter, age, number; char letter; float pi; boolean flag; //给以上变量赋值 counter = 3; number = counter; letter = 'z'; pi = 3.1415927 flag = true counter = 'z' number = 7.6 flag = 1;
3.10.9 A Shortcut, Declare and Assign at the Same Time
Often you will want to declare a variable and assign a value to it at the same time, you can do this as follows:
int counter = 0; boolean flag = false;