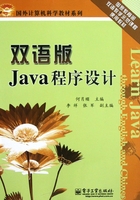
2.3 Write the First Java Program
A class definition has been created as a prototype. It can be used as a template for creating new classes that add functionality. Objects are programming units of a particular class. Dynamic loading implies that applications can request new objects of a particular class to be supplied on an “as needed” basis. Objects provide the extremely useful benefit of reusable code that minimizes development time.
对象是类的具体实现。动态加载意味着应用程序能够在需要的时候请求一个特殊类的实例。
A Java application resembles programs in most compiled languages. Code in object format resides on the user’s machine and is executed by a run-time interpreter that normally has to be user installed.
You wrote a simple ‘hello world!’ application to test the development environment. Now comes the explanation of the basic structure of Java applications using it as an example. Applications are stand-alone and are invoked (or executed) by using a Java interpreter.
/** * The HelloWorldApp 类实现一个应用 * 显示"Hello World!". */ public class HelloWorldApp { public static void main(String[] args) { System.out.println("Hello World!"); } }
The first four lines is a comment on the application’s function. Comments are not required but are extremely useful as documentation within the source. Other notes and doc files may get lost but these stay right where they are most useful. A long comment starts with a /* or /** and ends with a */ .Short one line comments begin with // and end with the <return>.
The fifth line starts with the reserved word public. This indicates that objects outside the object can invoke (or use) it. The reserved word class indicates that we are building a new object with the name that follows. HelloWorldApp is the object name (your choice) and is case sensitive. Java ‘style’is to capitalize the first letter of each word only. The line concludes with an opening curly bracket that marks the start of the object definition.
注释对于理解代码有很重要的作用,有单行注释和多行注释。public修饰符表示其他对象可以调用该程序。HelloWorldApp是一个类名字,而该类的类体包含在花括号内。
Line six is an invocation of an initialization method (or procedure in older languages). Static indicates that it calls the class and not an ‘instance’ of the class. The concept of ‘instance’ will be discussed later in the tutorials. The method’s name is main and the reserved word void indicates that no result is returned back. Main has arguments (aka parameters) in round brackets. String[] indicates the variable type is an array and args is a reserved word indicating that it is the command line values that are being passed over to main. The line finishes with an opening bracket for the main method.
main函数是该程序的主函数,也是程序执行的入口点。main函数没有返回类型,但是具有一个参数String数组,该数组可以接收来自控制台的输入参数。
Line eight invokes the println method of the system.out object. What is to be printed is passed in the argument as a string parameter. Note that each Java statement concludes with a semicolon.
Finally closing curly brackets are used for the main and for the object definition.