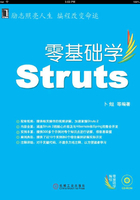
14.2 Spring的依赖注入
使用Spring的依赖注入可以为对象注入属性值。Spring的依赖注入分为两种,一种是通过Set方法注入依赖,一种是通过构造函数注入依赖。
14.2.1 通过Set方法注入依赖
下面来看如何通过Spring的依赖注入来为对象注入属性值。首先修改Student类,为其添加name属性以及name属性的setter和getter方法,代码如下所示。
package net.hncu.demo02; import net.hncu.demo01.Person; public class Student implements Person { //学生名字 private String name; //实现Person接口中的go方法 public void go(){ System.out.println( name + "去教室上课"); } //设置学生名字 public void setName(String name) { this.name = name; } //返回学生名字 public String getName() { return name; } }
修改Teacher类,为其添加name属性以及name属性的setter和getter方法,代码如下所示。
package net.hncu.demo02; import net.hncu.demo01.Person; public class Teacher implements Person{ //老师名字 private String name; //实现Person接口中的go方法 public void go(){ System.out.println( name + "去教室讲课"); } //设置老师名字 public void setName(String name) { this.name = name; } //返回老师名字 public String getName() { return name; } }
在Spring配置文件中创建Student和Teacher实例,并设置其name属性值,代码如下所示。
<? xml version="1.0" encoding="UTF-8"? > <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.0.xsd"> <! -- 创建Student实例,并设置其name属性值 --> <bean id="student" class="net.hncu.demo02.Student"> <property name="name"> <value>小强</value> </property> </bean> <! -- 创建Teacher实例,并设置其name属性值 --> <bean id="teacher" class="net.hncu.demo02.Teacher"> <property name="name"> <value>张老师</value> </property> </bean> </beans>
在主程序中取得Spring容器中的Teacher类和Student类实例,并调用实例的go()方法,代码如下所示。
package net.hncu.demo02; import net.hncu.demo01.Person; import org.springframework.beans.factory.xml.XmlBeanFactory; import org.springframework.core.io.ClassPathResource; public class Test { public static void main(String[] args) { //创建ClassPathResource实例 ClassPathResource isr = new ClassPathResource("beans2.xml"); //创建XmlBeanFactory实例 XmlBeanFactory factory = new XmlBeanFactory(isr); //从Spring容器中获得Teacher实例 Person teacher = (Person) factory.getBean("teacher"); teacher.go(); //从Spring容器中获得Student实例 Person student = (Person) factory.getBean("student"); student.go(); } }
运行该Java程序,在控制台中打印输出如下语句,代码如下所示。
张老师去教室讲课 小强去教室上课
14.2.2 引用其他Bean
有时候实例需要调用其他类的实例,这时可以不用直接调用实例。而是在Spring容器中创建该实例,并将该实例注入到需要调用它的实例中。
修改Teacher类,添加属性student以及student属性的setter和getter方法,代码如下所示。
package net.hncu.demo03; import net.hncu.demo01.Person; public class Teacher implements Person{ //老师名字 private String name; //老师最喜欢的一个学生 private Student student; //实现Person接口中的go方法 public void go(){ System.out.println( name + "去给" + student.getName()+ "讲课"); } //设置老师名字 public void setName(String name) { this.name = name; } //返回老师名字 public String getName() { return name; } //设置学生 public Student getStudent() { return student; } //返回学生 public void setStudent(Student student) { this.student = student; } }
在Spring配置文件中创建Student和Teacher实例,并设置其name属性值。还需要为Teacher实例设置其student属性值,可以使用ref标签直接引用当前IoC容器中的Student实例,代码如下所示。
<? xml version="1.0" encoding="UTF-8"? > <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.0.xsd"> <! -- 创建Student实例,并设置其name属性值 --> <bean id="student" class="net.hncu.demo03.Student"> <property name="name"> <value>小强</value> </property> </bean> <! -- 创建Teacher实例,设置其name属性值,设置其student属性值--> <bean id="teacher" class="net.hncu.demo03.Teacher"> <property name="name"> <value>张老师</value> </property> <property name="student"> <ref bean="student"/> </property> </bean> </beans>
在主程序中取得Spring容器中的Teacher类实例,并调用实例的go()方法,代码如下所示。
package net.hncu.demo03; import net.hncu.demo01.Person; import org.springframework.beans.factory.xml.XmlBeanFactory; import org.springframework.core.io.ClassPathResource; public class Test { public static void main(String[] args) { //创建ClassPathResource实例 ClassPathResource isr = new ClassPathResource("beans3.xml"); //创建XmlBeanFactory实例 XmlBeanFactory factory = new XmlBeanFactory(isr); //从Spring容器中获得Teacher实例 Person teacher = (Person) factory.getBean("teacher"); teacher.go(); } }
运行该Java程序,在控制台中打印输出如下语句。
张老师去给小强讲课
14.2.3 使用构造函数注入依赖
前面介绍了如何通过Set方法注入依赖,Spring还允许使用构造方法注入依赖。
修改Teacher类,为其添加构造函数,其中构造函数接受两个参数,分别为name和student,代码如下所示。
package net.hncu.demo04; import net.hncu.demo01.Person; public class Teacher implements Person{ //老师名字 private String name; //老师最喜欢的一个学生 private Student student; public Teacher(){ } //构造方法 public Teacher(String name, Student student) { this.name = name; this.student = student; } //实现Person接口中的go方法 public void go(){ System.out.println( name + "去给" + student.getName()+ "讲课"); } //设置老师名字 public void setName(String name) { this.name = name; } //返回老师名字 public String getName() { return name; } //设置学生 public Student getStudent() { return student; } //返回学生 public void setStudent(Student student) { this.student = student; } }
在Spring配置文件中创建Student实例,并设置其name属性值。创建Teacher实例,通过构造函数设置其name和student属性值,代码如下所示。
<? xml version="1.0" encoding="UTF-8"? > <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.0.xsd"> <! -- 创建Student实例,并设置其name属性值 --> <bean id="student" class="net.hncu.demo04.Student"> <property name="name"> <value>小强</value> </property> </bean> <! -- 创建Teacher实例,通过构造函数设置其name和student属性值--> <bean id="teacher" class="net.hncu.demo04.Teacher"> <constructor-arg> <value>张老师</value> </constructor-arg> <constructor-arg> <ref bean="student"/> </constructor-arg> </bean> </beans>
在主程序中取得Spring容器中的Teacher类实例,并调用实例的go()方法,代码如下所示。
package net.hncu.demo04; import net.hncu.demo01.Person; import org.springframework.beans.factory.xml.XmlBeanFactory; import org.springframework.core.io.ClassPathResource; public class Test { public static void main(String[] args) { //创建ClassPathResource实例 ClassPathResource isr = new ClassPathResource("beans3.xml"); //创建XmlBeanFactory实例 XmlBeanFactory factory = new XmlBeanFactory(isr); //从Spring容器中获得Teacher实例 Person teacher = (Person) factory.getBean("teacher"); teacher.go(); } }
运行该Java程序,在控制台中打印输出如下语句。
张老师去给小强讲课